Functions in ClojureScript¶
So, how do you call functions in ClojureScript? There’s a universal rule: Write an open parenthesis, the name of the function, the function’s arguments, and a closing parenthesis.
Doing Arithmetic with Functions¶
Bringing back the addition function box from the previous page:
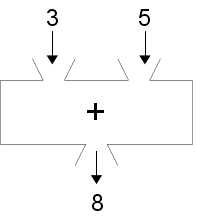
Addition as a function box
If you want to add 3 and 5 in ClojureScript, you write the following expression: an open parenthesis, the name of the function (in this case, its name is +
), the arguments, and the closing parenthesis.
In keeping with the philosophy of this book, you didn’t merely add 3 and 5, you transformed the numbers 3 and 5 into 8 by applying the add function to them. OK, so how would you write an expression that applies the multiply function to the numbers 8 and 9 to get their product? Or, in more ordinary terminology, write an expression that uses the *
function to multiply 8 by 9.
Try it in the active code box below. (Note: the line beginning with ;
is a comment. Comments are for us humans to read; the computer ignores the semicolon and everything
that follows it on that line.)
In both these cases, it doesn’t matter which order you put the numbers, since addition and multiplication
are commutative (a fancy math term for “order doesn’t matter”). But what about division and subtraction, where order does matter? Which number comes first? Try doing a function call using the /
function to divide 8 by 2. Experiment with both orders to see which one gives you the correct answer of 4.
quot
function. To get the remainder after integer division, use the rem
function. Thus, (quot 35 4)
is 8, and (rem 35 4)
is 3.
The arithmetic functions can take more than two arguments, so if you need to multiply 7 times 6 times 23, you can write (* 7 6 23)
; similarly, (+ 10 4 2 11)
will result in 27. However, what happens if you need to do arithmetic involving more than one operation, such as 3 + 4 * 5? Go to the next page to find out how to do that.